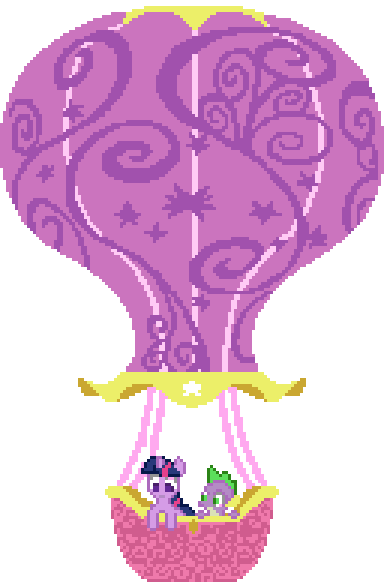
Try pressing the WASD keys
Playing the game
If you are just looking to play the game, you can download the rom by clicking the button above, or from my downloads page.
If you want to take a look at the source code (and all previous roms) check out my github. https://github.com/YellowTeamRobot/RainbowDashEmUp
I personally use the mGBA emulator for Gameboy Advance emulation, links to their github and website, but it should work on any popular GBA emulator.
I have also tested the game on physical hardware (GBA, GBA SP, DS) using an EZ-Flash Omega, and can confirm that it works as expected.
The Development Process
I’ve been meaning to post a summary of the development process for this game for a while, and now I have finally decided to do so.
How it Started
At some point I had the idea of making a game for the GBA. I looked into how I would go about doing this, but that didn’t go very far. Perhaps the extent of this knowledge was that I knew it was typically programmed with C, and that it was easier than programming for a DS.
In the Fall of 2021, I took a college course called Computer Organization. The course was aimed at learning how computers worked, and learning about their architecture and how they are set up. Since this course focused on learning about how the hardware (mainly CPU) worked, we also spent a significant time learning both C and x86 Assembly, since they’re designed to work super close to the hardware.
Because we were learning C and Assembly, and I knew that the GBA was coded using C and Assembly, I decided that would be the perfect opportunity to learn about the GBA, and make a game both as a personal project, and as a final project for the class.
Learning the Ropes
First thing first, I needed to learn C. Having coded in a variety of languages before, most (if not all) of which are at least somewhat based on C, picking up the basics of C was as simple as learning the syntax. Obviously there were some new things I had to learn, like using pointers, but through class and through hands on experience, I got the hang of these things. Coincidentally, we usually happened to talk about things in class right when I found I needed to use/learn about them, which was quite nice.
Learning and improving my knowledge of C was an ongoing process during this, but I wanted to code for the GBA. Luckily, devkitPRO provides most of the tools needed for compiling a GBA rom. After setting up everything to allow me to work with those tools directly from Visual Studio Code, I started looking for resources to learn about how to actually code for the GBA.
I found a great many things, but it seemed that the best resource both for learning how to program for the GBA, and as a good library, was Tonc (LibTonc). http://coranac.com/tonc/text/ Following some of the basics from this, along with looking at examples from other resources, I started learning.
My first major breakthrough was drawing a single magenta pixel on the screen. It doesn’t sound like much, but it was a very important milestone for me, now I could display things on the screen. (To Note, I was only using bitmap videomodes at this point)
Now that I could draw things on the screen, what should make? I decided to make a Breakout-like game, as I knew it would be simple enough, and wouldn’t have any surprises I’d need to figure out.
This is what I ended up with. (Don’t mind the gif compression)
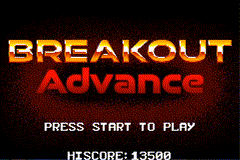
During this time, I also played around with creating a program that would display every single color the GBA can display, without any repeats, and doing so in a nice looking manner. And I ended up with something that looked like this.
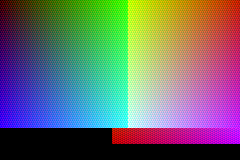
I also played around a bit with the notion of creating a sort of clone or de-make of the DOS game Scorched Earth, because I always loved that game. I didn’t go through with the entire thing, and it was more of a glorified tech demo that was an excuse for myself to see how much unoptimized trigonometry the GBA could handle, but I created terrain generation, a moving tank, projectiles, and terrain deformation/explosions (I also figured out how to do some sound, although it was nothing fancy, just some generated noise to resemble an explosion).
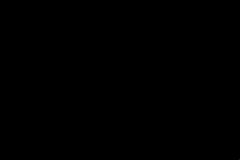
At this point I had fulfilled my goal, I made a GBA game, a GBA version of Breakout. It also filled all the criteria for the final project, so I could have just been done with the entire thing, and submitted what I had. But I wasn’t satisfied. I could have gone along and finished my version of Scorched Earth, but it was lacking something that most official GBA games made use of. Sprites.
Up until this point, I had been using bitmap video modes on the GBA, but most official games don’t use these modes because they are slow. The GBA has specific Sprite and Tile based video modes which use some clever tricks to run much faster, and this is what most games used, and is what gives most games of the time their signature look. I wanted to learn how to use sprites, so that is exactly what I did.
Sprites and My Little Pony
So I knew that I was going to make something with sprites, but before you make something, you need to have some idea what you are going to make. I did some brainstorming and came up with a number of ideas. One idea was making a version of the puzzle game Columns for the GBA, and keeping the original art style. However, I didn’t do this because Columns Crown, although having a different art style than the original, did already exist on the GBA. I also contemplated making a version of the NES puzzle game Yoshi, but I ended up not doing so because, to be honest, I have never been able to wrap my head around how to code the logic for puzzle games (this was another reason why I did not make Columns). I was also thinking of making a platformer, but I knew it would take a lot of work to get the various systems set up, not to mention needing to design multiple levels and creating a lot of art. My final idea was a side-scrolling shooter, or shootemup, which was similar enough to a platformer in that it is 2D side-scrolling, but without setting up all the physics or needing extensive level design. All I needed now was a theme.
Sometime during the semester, someone convinced me to watch the newly released My Little Pony: A New Generation movie on Netflix. I like watching cartoons and animated movies in general, and I actually enjoyed the movie. One thing leads to another, and I found myself watching the MLP Friendship is Magic series, which I had grown up around, but never watched. It was cute, colorful, and wholesome, with comedy, pop-culture references, and a decent plot. I really ended up enjoying it because of this, it was something different, more lighthearted and wholesome, that I didn’t see in many other shows. So of course I had the idea to make it MLP themed.
This provided me with a number of things to work off of. First, with MLP:FiM being as popular as it was, I was able to find some character sprites I could use that someone else had already made. (Big thanks to My Little Gauntlet for creating the Rainbowdash sprites, and letting others use them). Second, because it’s a TV show, I had plenty of material to work with and reference when creating all the other art assets in the game. Third, I found a way to incorporate the theme into the game rather well. Rainbowdash was a weather pony, so I made the goal of the game to have her get rid of rainclouds. Being in the sky also provided a perfect opportunity to make a parallax background, with multiple layers of clouds scrolling past.
So now that I knew what I wanted to make, it was time to get to work.
First I had to get the sprite on the screen. Following Tonc, I used a tool (Usenti) to convert the spritesheet into a format useable by the GBA. Then I had to load that spritesheet into memory, and finally draw it on screen. After that, I just added a loop to go through the animation, and some code to change the sprite position when the d-pad is pressed and I got this.

I also added in a blink animation.
Now that I had a handle on sprites, it was time to figure out backgrounds. First thing obviously was to make the backgrounds, so I found a lot of pictures of MLP clouds online, and created three layers of clouds. One with the base sky color and a few distant cloud silhouettes, another closer one with some regular clouds, and the closest layer which consisted of a big mass of cloud. The GBA has 4 background “layers” that can be used at a time, and moved independently of one another, so all I had to do was put the image on its corresponding layer, and then set how fast it should scroll. I also added some vertical parallax, where the closer layers move up/down more than the further layers when the character moves up or down. That looked like this (image does not show vertical parallax).
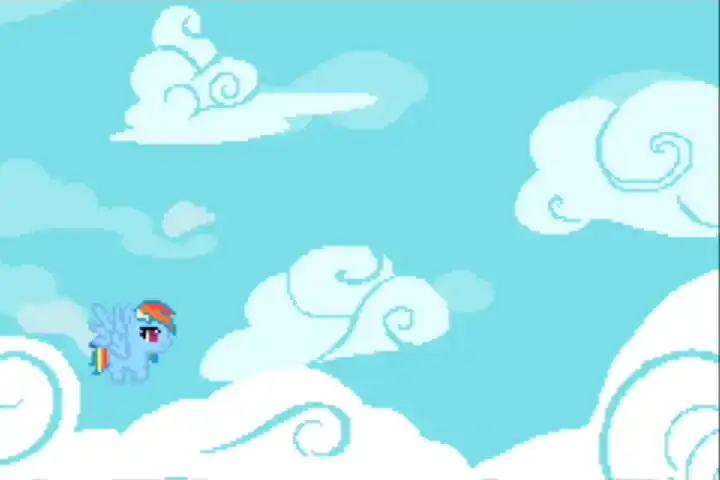
I still had one background layer left, so I wanted to do something interesting with it. I could have just used it to display score and text, but since I wasn’t going to be using many sprites on screen at a time, I could just handle that with sprites instead of using a background layer. I decided that I would have Twilight’s hot-air balloon float by every once in a while, so I used the fourth background layer for this. However, upon implementing it, this happened.
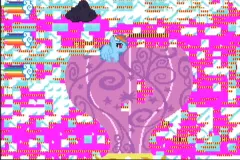
What was happening was that when I added this fourth set of data to the memory for the backgrounds, it was too big, and ended up overwriting other background data. Obviously we don’t want this to happen, so I worked on paring down all the backgrounds, reusing specific tiles where I could so that everything fit into the memory. After a few hours of editing the backgrounds, I finally got it to fit exactly in the memory allocated for backgrounds, and keeping everything looking nice.
Mechanics and Additional Visuals
At this point, the clouds scrolled nicely in the background, and the balloon would float by every once in a while, slowly bobbing up and down. Somewhere during this process I also added some other sprites to the game, but I had not done anything with them yet. In the above picture, you can see them just chilling on the screen. So the next thing to do was to add movement and collisions for the rainclouds and projectiles. The collision handling is very simplistic, it just loops through every projectile/raincloud on screen, and checks if they any of their corners overlap. So now I had this.
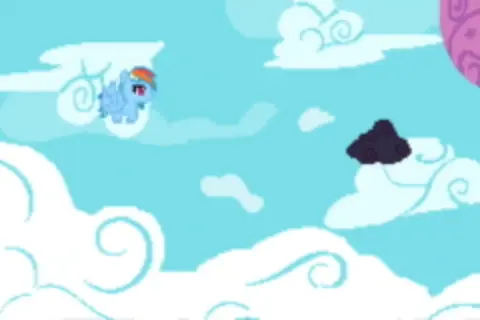
After this I worked on creating the main menu, along with creating a better looking logo for the title screen.
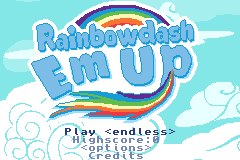
At this point I went ahead and added the score text and lives counter, added a boss mechanic, and for the rest of the development, I slowly added more cloud formations to choose from. However, there was one thing that was lacking, sound.
Do You Hear That? (Not Yet)
I mentioned earlier that I had dabbled in a little bit of sound when working on making the prototype for a Scorched Earth game. I had to make heavy reference of the GBA technical manual, GBATEK, which I found at http://www.problemkaputt.de/gbatek-gba-technical-data.htm. I think that Tonc had a few sound features already built in, mainly generating sine wave sounds, but I had to do some extra tinkering to generate noise. But even with this, I could only create very basic sounds reminiscent of rather old arcade machines, and I knew the GBA was capable of proper sound reproduction, even if it was compressed. At the moment, I could create sound effects like this.
However, thanks to the generosity of James Daniels, the Apex Audio System was released for anyone to use. https://github.com/stuij/apex-audio-system. After learning how this worked, I was finally able to have proper sound in my game. I found an instrumental version of the MLP theme online, and edited it to loop perfectly. My intention was for this to be the main menu music, and to have a few original tracks for the actual gameplay, however, that didn’t pan out, so I just used the one song for the entire game. I then worked on creating some sound effects for various things, but I was still missing something. Voicelines.
This wasn’t a problem however, since thanks to the marvels of modern technology, speech synthesis has become remarkably better than it once was. One specific text-to-speech algorithm that is beyond impressive is the one over at 15.ai. This of course had the added benefit of having MLP characters as some of the best voices available, as they were sort of the foundation for the “emotive speech” that makes this TTS better than something like Tacotron. I find things like this to be really interesting, and definitely recommend checking it out, and even reading the paper on it (once it’s actually written). With all that said, I can now reproduce audio like this (albeit with some compression).
I Thought You Mentioned Assembly
During the process of creating this game, I not only worked in C, I also worked in Assembly, specifically, ARM Assembly for the ARM7tdmi processor. In class, we were learning x86 assembly, and quite frankly, I only paid as much attention to it as I needed to, because I was more interested in ARM assembly. That being said, I did pay enough attention to learn the basics, even if it ended up being different. Using what I learned in class, along with what I learned from, https://patater.com/gbaguy/gbaasm.htm and also referencing this pdf (which I have on my website for archival/reference purposes). I also learned how it worked by analyzing some examples on gbadev.org.
After a bit of work, I created a few assembly functions to load and save numbers in the save ram, which I used to store high scores. It was rather basic, but it works.
The End of My Journey
Anyways, that about sums it all up. I’m sure I forgot to mention some things that I wanted to, but at the end of the day, I finished the game. If you read until the end, congratulations. As a reward, you get to learn about some fun extras. Try pressing B along with either L, R, Up, Down, Left, or Right, while on the main menu. Or, when playing the game, try using the Konami Code.
That’s all Folks!
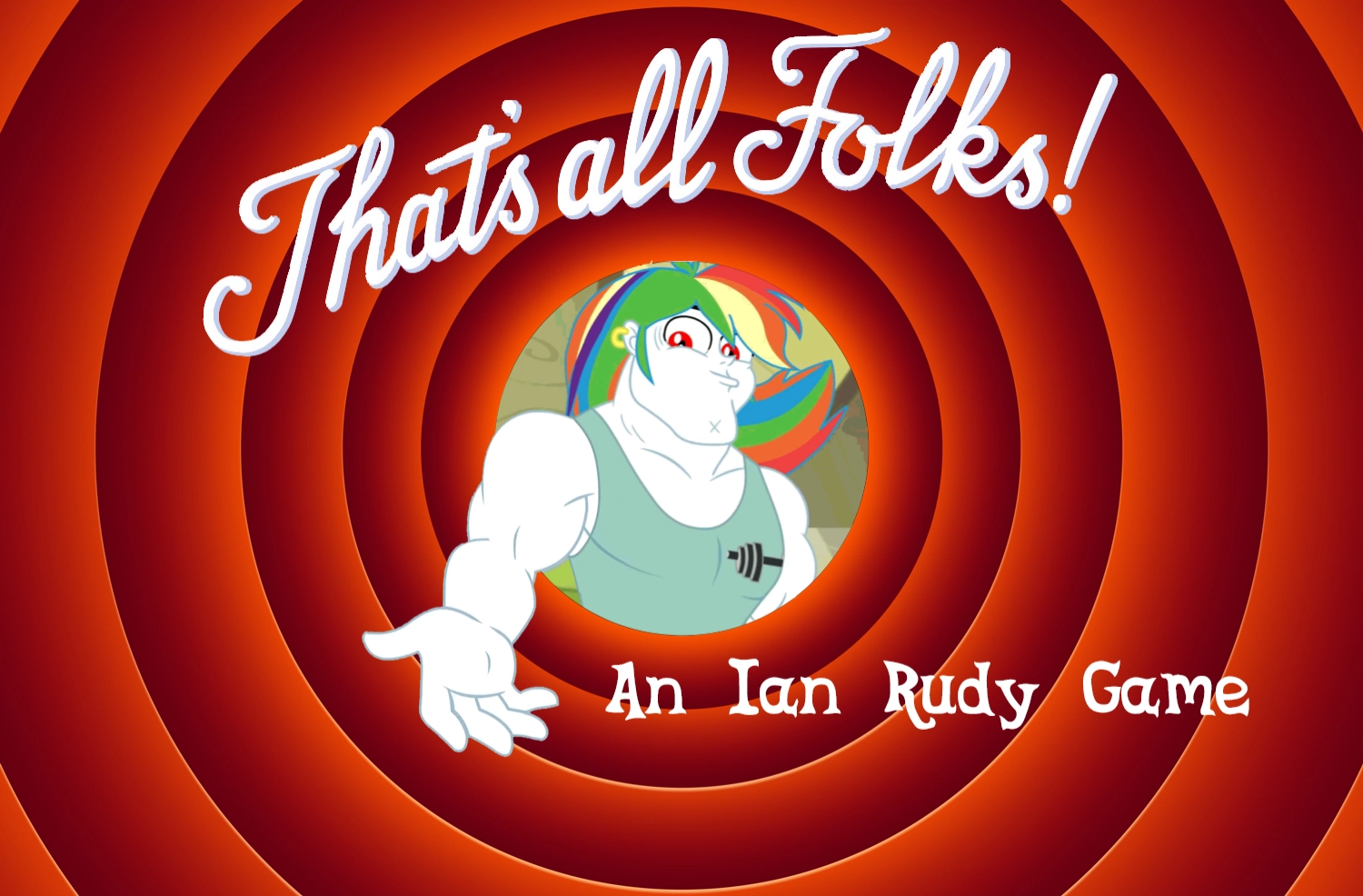